
Introduction
Getting the current time in UTC (Coordinated Universal Time) is essential in various applications, including programming, international business, and scientific research. UTC serves as the primary time standard for modern civilization, providing a consistent and reliable way to coordinate clocks worldwide. In this article, we will explore five ways to obtain the current time in UTC, covering different programming languages and approaches.

Method 1: Using JavaScript
JavaScript is a versatile programming language used extensively in web development. To get the current time in UTC using JavaScript, you can leverage the Date object and its getUTCHours(), getUTCMinutes(), and getUTCSeconds() methods.
javascript const now = new Date(); const utcTime = ${now.getUTCHours()}:${now.getUTCMinutes()}:${now.getUTCSeconds()}; console.log(utcTime);
This code snippet creates a new Date object representing the current time and extracts the UTC hours, minutes, and seconds using the corresponding methods. The results are then concatenated to form a string representing the current time in UTC.

Method 2: Using Python
Python is a popular programming language used in various domains, including data science, machine learning, and web development. To get the current time in UTC using Python, you can use the datetime module and its utcnow() function.
python import datetime
utctime = datetime.datetime.utcnow().strftime("%H:%M:%S") print(utctime)
This code snippet imports the datetime module and uses the utcnow() function to get the current time in UTC. The strftime() method is then applied to format the time as a string in the format HH:MM:SS.

Method 3: Using Java
Java is an object-oriented programming language used extensively in Android app development, web development, and enterprise software development. To get the current time in UTC using Java, you can use the java.time package and its Instant class.
java import java.time.Instant;
public class Main { public static void main(String[] args) { Instant instant = Instant.now(); System.out.println(instant); } }
This code snippet imports the java.time package and uses the Instant class to get the current time in UTC. The now() method returns an Instant object representing the current time, which is then printed to the console.
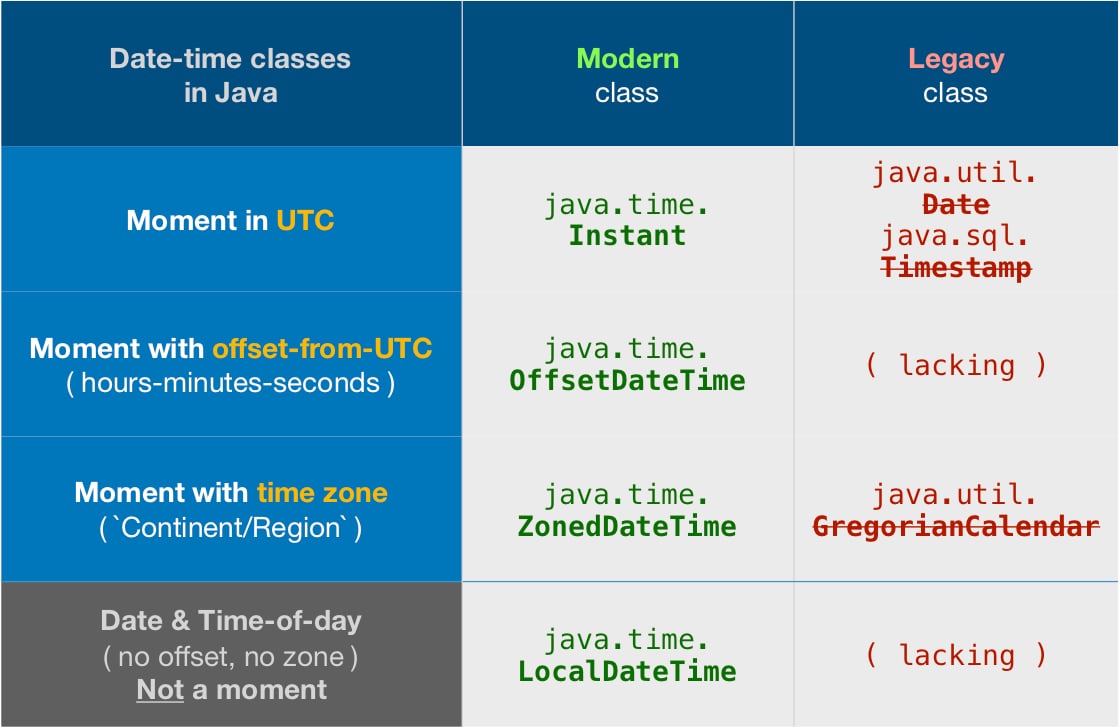
Method 4: Using the date Command
The date command is a Unix command used to display the current time and date. To get the current time in UTC using the date command, you can use the following syntax:
bash date -u +%H:%M:%S
This command displays the current time in UTC, formatted as HH:MM:SS.

Method 5: Using an Online World Clock
An online world clock is a website or web application that displays the current time in different time zones around the world. To get the current time in UTC using an online world clock, you can simply visit a website such as WorldTimeBuddy or TimeAndDate and select the UTC time zone.

In conclusion, there are several ways to get the current time in UTC, including using programming languages such as JavaScript, Python, and Java, as well as using the date command and online world clocks. Each method has its own advantages and disadvantages, and the choice of method depends on the specific use case and requirements.
We hope this article has provided you with a comprehensive overview of the different ways to get the current time in UTC. If you have any questions or need further clarification, please don't hesitate to ask.
FAQs:
What is UTC time?
+UTC (Coordinated Universal Time) is the primary time standard for modern civilization, providing a consistent and reliable way to coordinate clocks worldwide.
Why is UTC time important?
+UTC time is essential in various applications, including programming, international business, and scientific research, as it provides a standardized way to coordinate clocks worldwide.
What are the different ways to get the current time in UTC?
+There are several ways to get the current time in UTC, including using programming languages such as JavaScript, Python, and Java, as well as using the date command and online world clocks.
Gallery of 5 Ways To Get The Current Time In Utc



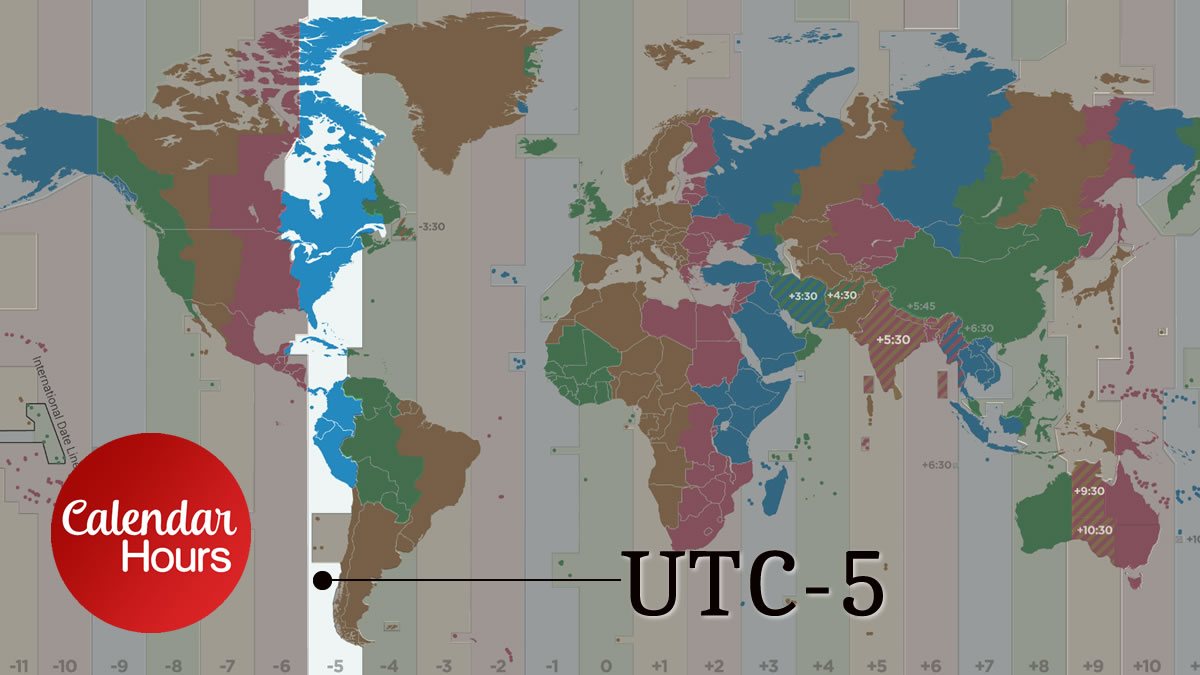
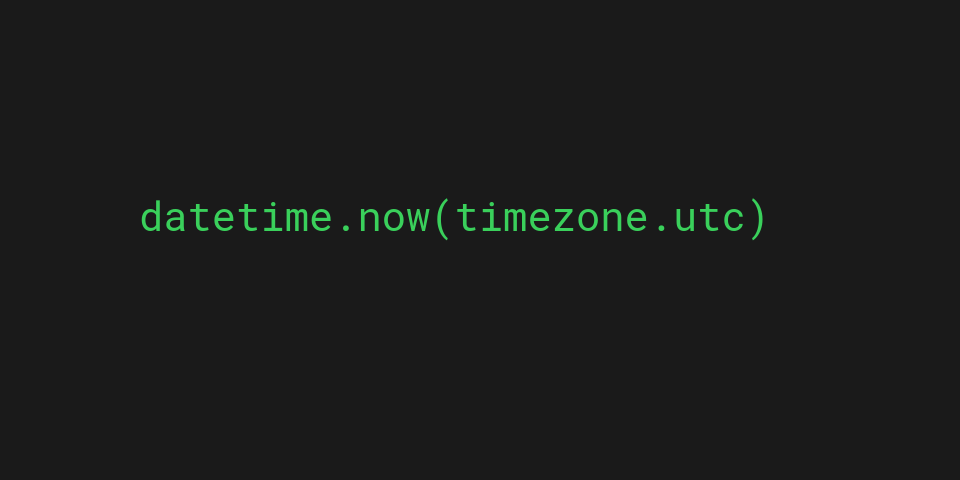
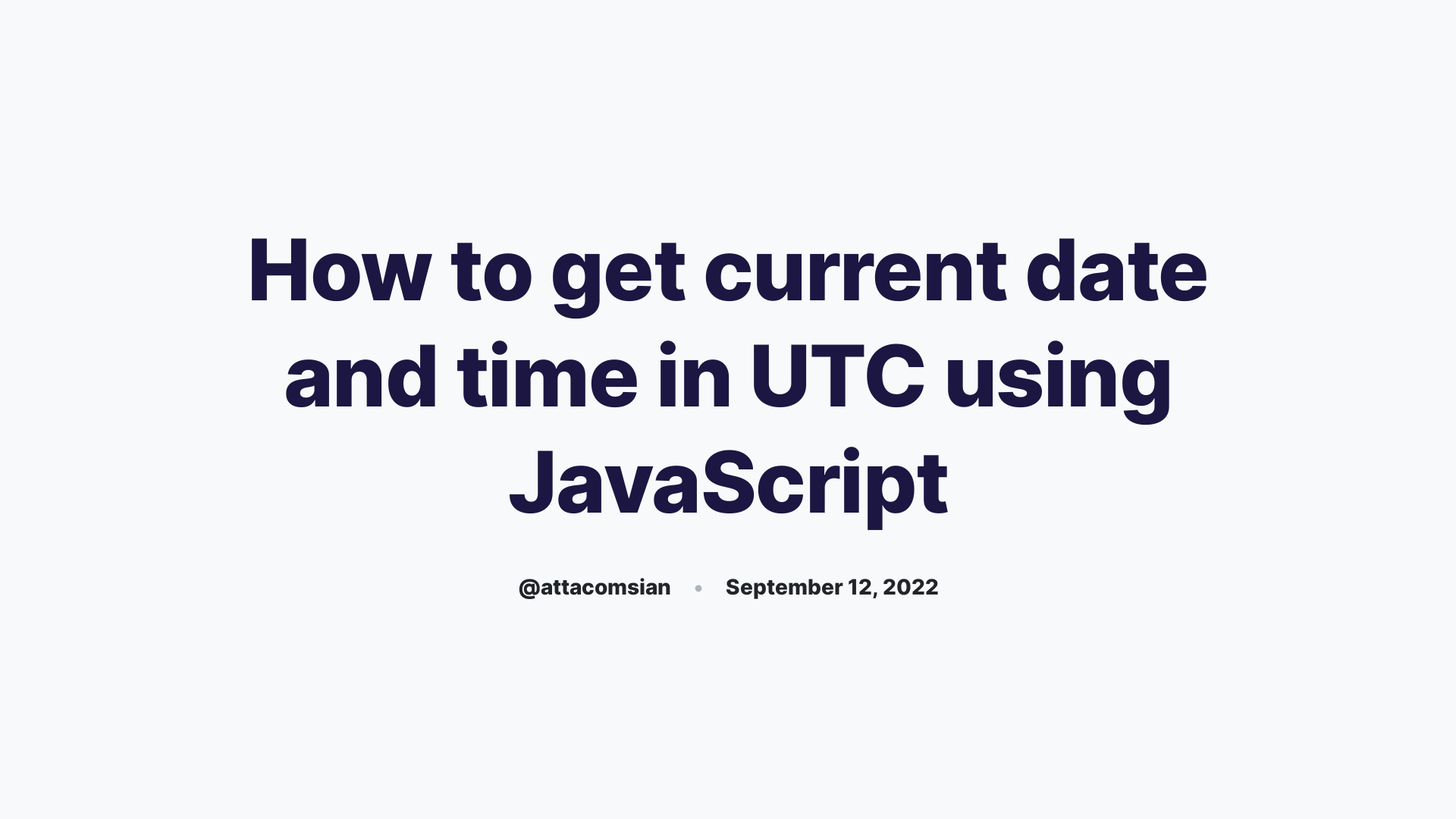
